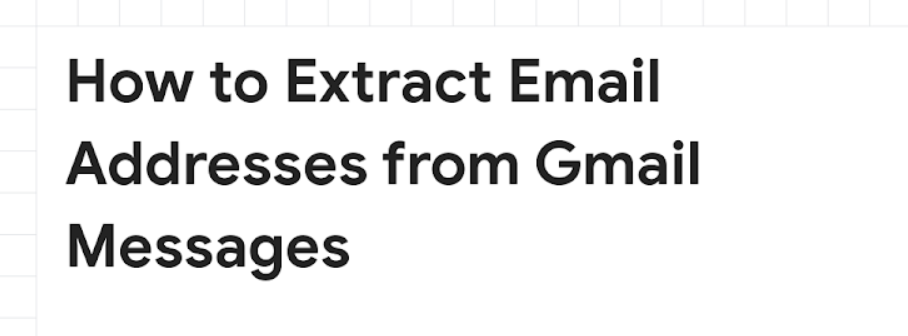
Method 1: Using Google Apps Script
- Open Google Sheets:
- Create a new Google Sheet.
- Open Script Editor:
- Go to
Extensions
>Apps Script
.
- Go to
- Copy and Paste the Script:
- Copy the following script and paste it into the script editor. This script will iterate through all threads in your Gmail account and extract email addresses from the sender and recipients.
- Run the Script:
- Save the script and click the play button (Run) to execute the function
getAllEmails
. It will start extracting email addresses from all folders in your Gmail account and populating them in the Google Sheet.
function getAllEmails() {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var emailAddresses = [];
var row = 1;
// Get all user labels
var labels = GmailApp.getUserLabels();
// Get all inbox threads separately
var inboxThreads = GmailApp.getInboxThreads();
// Function to process threads
function processThreads(threads) {
for (var j = 0; j < threads.length; j++) {
var messages = threads[j].getMessages();
for (var k = 0; k < messages.length; k++) {
var from = messages[k].getFrom();
var to = messages[k].getTo();
var cc = messages[k].getCc();
var bcc = messages[k].getBcc();
var emails = extractEmails(from).concat(extractEmails(to), extractEmails(cc), extractEmails(bcc));
emails.forEach(function(email) {
if (emailAddresses.indexOf(email) === -1) {
emailAddresses.push(email);
sheet.getRange(row, 1).setValue(email);
row++;
}
});
}
}
}
// Process inbox threads
processThreads(inboxThreads);
// Process threads in each user label
for (var i = 0; i < labels.length; i++) {
var threads = labels[i].getThreads();
processThreads(threads);
}
}
function extractEmails(str) {
var emails = [];
if (str) {
var matches = str.match(/[\w.-]+@[\w.-]+\.\w+/g);
if (matches) {
emails = matches;
}
}
return emails;
}
Code language: JavaScript (javascript)
The script exceeding the maximum execution time indicates that it is taking too long to process all the threads and messages in your Gmail account. To handle this, you can modify the script to process the emails in batches and use a script trigger to resume processing where it left off.
Modified Script to Process Emails in Batches:
- Store Progress:
- Use the Properties Service to store progress between executions.
- Batch Processing:
- Process a limited number of threads per execution to avoid exceeding the execution time limit.
- Reschedule Execution:
- Use a trigger to schedule the next batch if there are more threads to process.
function getAllEmails() {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var properties = PropertiesService.getScriptProperties();
var emailAddresses = properties.getProperty('emailAddresses') ? JSON.parse(properties.getProperty('emailAddresses')) : [];
var row = properties.getProperty('row') ? parseInt(properties.getProperty('row')) : 1;
var labelIndex = properties.getProperty('labelIndex') ? parseInt(properties.getProperty('labelIndex')) : 0;
var threadIndex = properties.getProperty('threadIndex') ? parseInt(properties.getProperty('threadIndex')) : 0;
var processedThreads = 0;
var BATCH_SIZE = 100; // Number of threads to process per execution
// Get all user labels
var labels = GmailApp.getUserLabels();
// Get all inbox threads separately
var inboxThreads = GmailApp.getInboxThreads();
// Function to process threads
function processThreads(threads) {
for (var j = threadIndex; j < threads.length && processedThreads < BATCH_SIZE; j++, processedThreads++) {
var messages = threads[j].getMessages();
for (var k = 0; k < messages.length; k++) {
var from = messages[k].getFrom();
var to = messages[k].getTo();
var cc = messages[k].getCc();
var bcc = messages[k].getBcc();
var emails = extractEmails(from).concat(extractEmails(to), extractEmails(cc), extractEmails(bcc));
emails.forEach(function(email) {
if (emailAddresses.indexOf(email) === -1) {
emailAddresses.push(email);
sheet.getRange(row, 1).setValue(email);
row++;
}
});
}
}
threadIndex = j;
}
// Process inbox threads
if (labelIndex == 0) {
processThreads(inboxThreads);
}
// Process threads in each user label
for (; labelIndex < labels.length && processedThreads < BATCH_SIZE; labelIndex++) {
var threads = labels[labelIndex].getThreads();
processThreads(threads);
if (threadIndex < threads.length) {
break;
} else {
threadIndex = 0;
}
}
// Store progress
properties.setProperty('emailAddresses', JSON.stringify(emailAddresses));
properties.setProperty('row', row);
properties.setProperty('labelIndex', labelIndex);
properties.setProperty('threadIndex', threadIndex);
// Reschedule if there are more threads to process
if (labelIndex < labels.length || threadIndex < inboxThreads.length) {
ScriptApp.newTrigger('getAllEmails')
.timeBased()
.after(1 * 60 * 1000) // Run after 1 minute
.create();
} else {
// Clear properties after completion
properties.deleteAllProperties();
}
}
function extractEmails(str) {
var emails = [];
if (str) {
var matches = str.match(/[\w.-]+@[\w.-]+\.\w+/g);
if (matches) {
emails = matches;
}
}
return emails;
}
Code language: JavaScript (javascript)