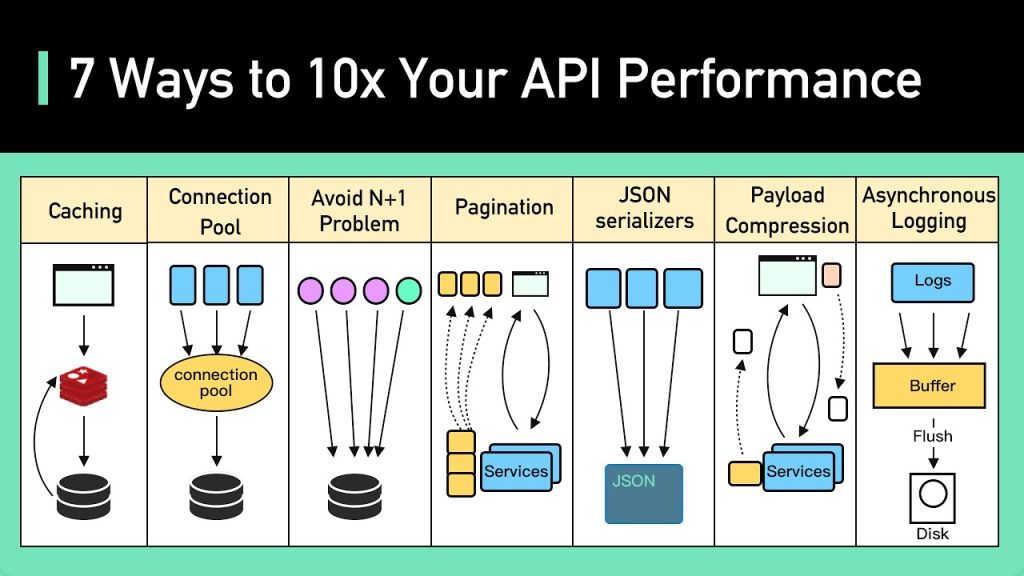
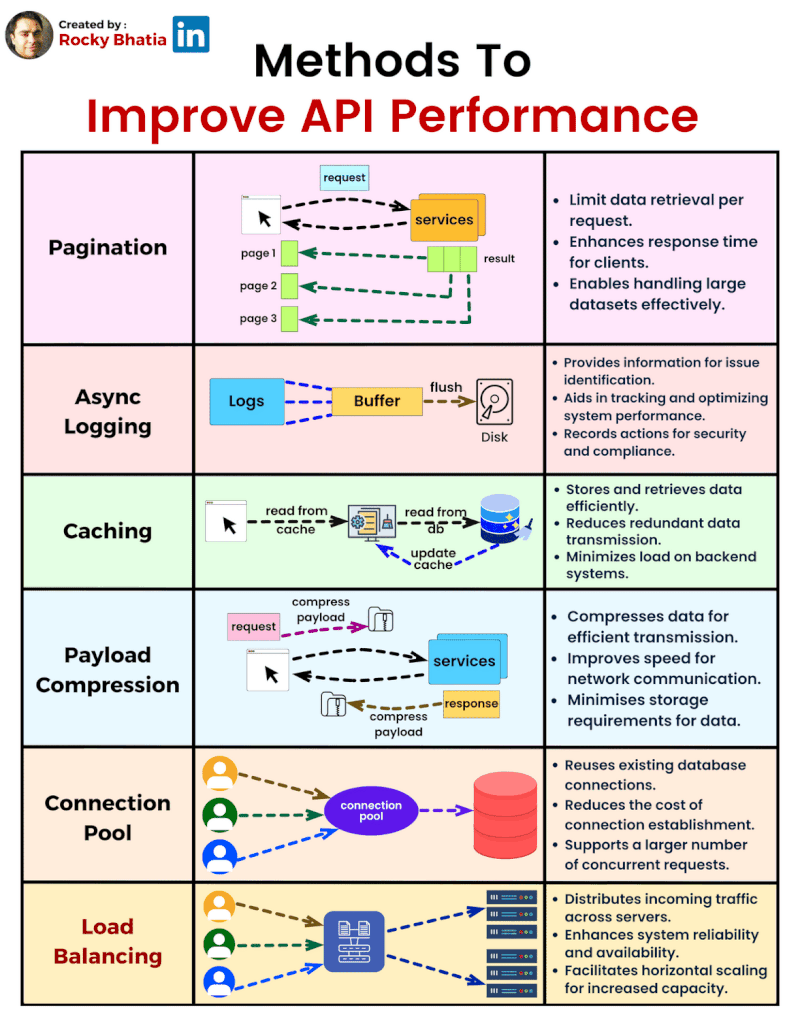
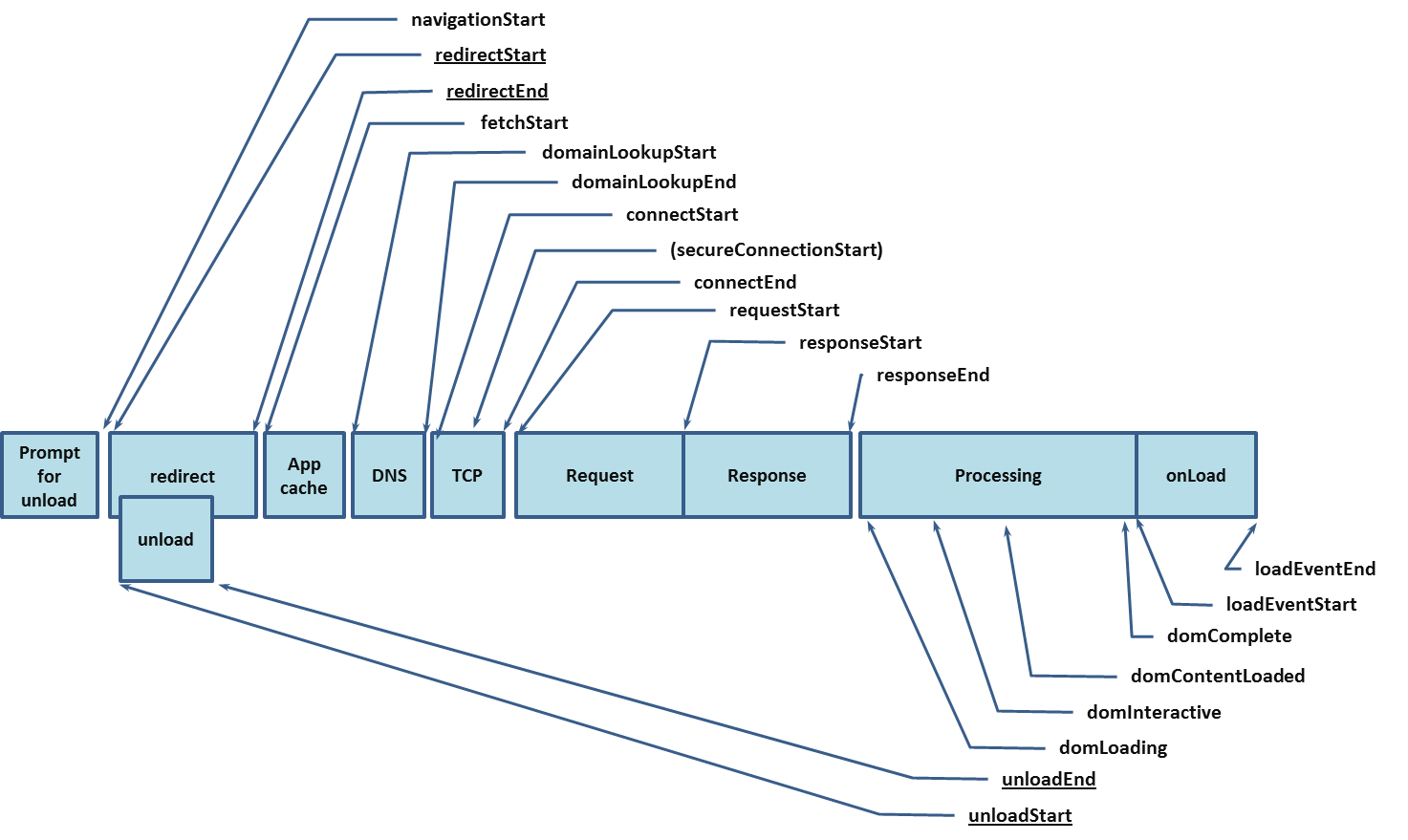
Improving API performance is crucial for creating efficient and responsive applications. Here’s a comprehensive guide to various methods for optimizing API performance:
1. Implement Caching
Caching is one of the most effective ways to improve API performance by storing frequently accessed data for quick retrieval.
Types of Caching:
- In-memory caching: Using tools like Redis or Memcached
- HTTP caching: Utilizing cache-control headers
- CDN caching: For geographically distributed content delivery
Implementation:
- Use cache annotations in your code (e.g., @Cacheable in Spring)
- Set appropriate cache expiration times
- Implement cache invalidation strategies
Example (Java with Spring):
java@Cacheable("users")
@GetMapping
public List<User> getAllUsers() {
return userRepository.findAll();
}
2. Optimize Database Queries
Efficient database interactions are crucial for API performance.
Strategies:
- Indexing: Create indexes on frequently queried fields
- Query optimization: Rewrite complex queries for efficiency
- Avoid N+1 queries: Use eager loading or batch fetching
Example:
sql-- Before
SELECT * FROM users WHERE email = 'example@example.com';
-- After (with index)
CREATE INDEX idx_email ON users(email);
3. Implement Compression
Compressing API responses reduces payload size and improves transfer speeds.
Methods:
- GZIP compression: Widely supported and effective
- Brotli: More efficient than GZIP but with less browser support
Implementation:
text# Spring Boot application.properties
server.compression.enabled=true
server.compression.mime-types=application/json,application/xml
server.compression.min-response-size=1024
4. Use Pagination and Filtering
These techniques help manage large datasets efficiently.
Pagination strategies:
- Offset-based: Using ‘limit’ and ‘offset’ parameters
- Cursor-based: Using a unique identifier for the last item
Filtering:
- Allow clients to specify required fields
- Implement server-side filtering to reduce data transfer
Example:
textGET /api/users?page=1&limit=50&fields=name,email
5. Implement Asynchronous Processing
Asynchronous APIs can handle multiple requests concurrently, improving overall throughput.
Techniques:
- Use non-blocking I/O
- Implement webhooks for long-running processes
- Utilize message queues for background processing
Example (Java):
java@GetMapping("/async")
public CompletableFuture<List<User>> getAllUsersAsync() {
return CompletableFuture.supplyAsync(() -> userRepository.findAll());
}
6. Optimize Payload Size
Reducing the amount of data transferred improves API speed.
Strategies:
- Remove unnecessary fields from responses
- Use efficient data formats (e.g., Protocol Buffers)
- Implement partial response techniques
Example:
java@GetMapping("/users")
public List<UserDTO> getUsers(@RequestParam(required = false) List<String> fields) {
List<User> users = userRepository.findAll();
return users.stream()
.map(user -> createDTO(user, fields))
.collect(Collectors.toList());
}
7. Use Connection Pooling
Reusing database connections reduces overhead and improves performance.
Implementation:
- Configure connection pooling in your database driver
- Adjust pool size based on your application’s needs
Example (HikariCP configuration):
textspring.datasource.hikari.maximum-pool-size=10
spring.datasource.hikari.minimum-idle=5
8. Implement Rate Limiting
Rate limiting prevents API abuse and ensures fair usage.
Strategies:
- Token bucket algorithm
- Fixed window counter
- Sliding window log
Example (Using a third-party library):
java@RestController
public class UserController {
@GetMapping("/users")
@RateLimit(limit = 100, duration = 60)
public List<User> getUsers() {
// Implementation
}
}
9. Use HTTP/2
HTTP/2 offers several performance improvements over HTTP/1.1.
Benefits:
- Multiplexing: Multiple requests over a single connection
- Header compression
- Server push
Implementation:
Configure your web server to use HTTP/2 (e.g., in Nginx or Apache)
10. Implement Proper Error Handling
Efficient error handling improves API reliability and helps in quick problem resolution.
Best Practices:
- Use appropriate HTTP status codes
- Provide detailed error messages
- Implement global exception handling
Example (Spring Boot):
java@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<?> handleResourceNotFoundException(ResourceNotFoundException ex) {
ErrorResponse error = new ErrorResponse("NOT_FOUND", ex.getMessage());
return new ResponseEntity<>(error, HttpStatus.NOT_FOUND);
}
}
By implementing these strategies, you can significantly improve your API’s performance, leading to faster response times, reduced server load, and improved user experience. Remember to continuously monitor and test your API to identify and address performance bottlenecks as they arise.